介绍
「编译原理」课程项目——利用 ANTLR4 工具,为 MiniJava 语言构造一个简易的编译器前端,将输入转化为抽象语法树,并进行一定的语义检查。
项目代码已开源在 GitHub。
语法
参考 Christoph Mallon, Jo ̈rg Herter. MiniJava Language Specification, October 21, 2009. 附件
功能
- 词法分析
- 语法分析
- 抽象语法树GUI
- 出错位置和类型提示
- 标识符定义检查——是否使用未定义标识符,是否重复定义标识符等
- 方法调用参数检查
- 方法返回类型检查
- 标识符类型检查
- 运算类型检查
语法描述
MiniJava.g4
1 | grammar MiniJava; |
软件说明
环境
- JDK 1.6
- Apache Maven 3.0.5
编译
mvn clean package assembly:single
运行
java -jar MiniJava-1.0-SNAPSHOT-jar-with-dependencies.jar [options...] files...
参数
1 | java -jar {jarFile} [options...] files... |
运行样例
命令
java -jar MiniJava-1.0-jar-with-dependencies.jar –gui sample.txt
输入
sample.txt
1 | class MyClass extends MyClass { |
输出
1 | ============== Analyzing '/Users/Yuanzi/Documents/project/MiniJava/src/main/resources/sample.txt' ...... |
GUI
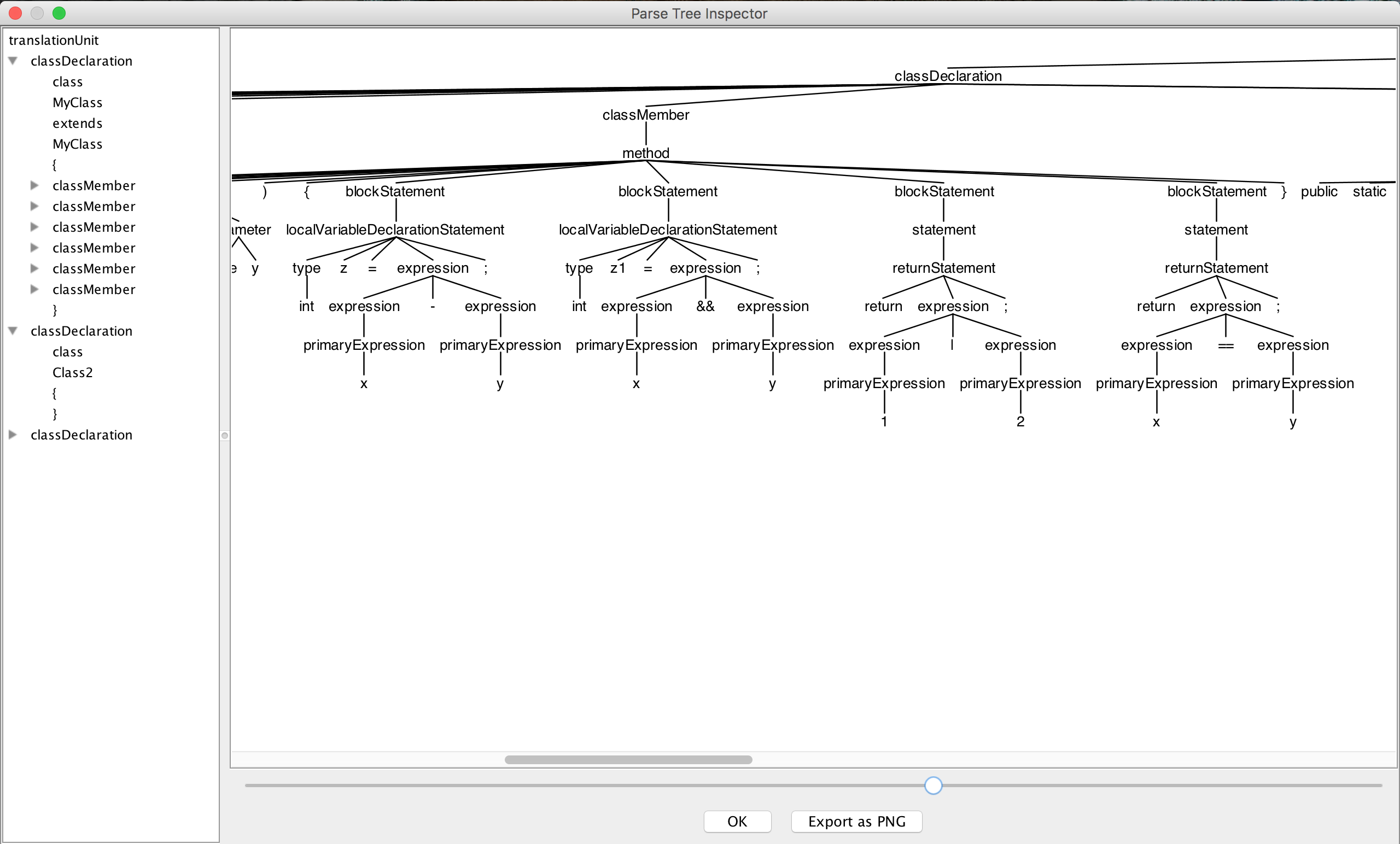